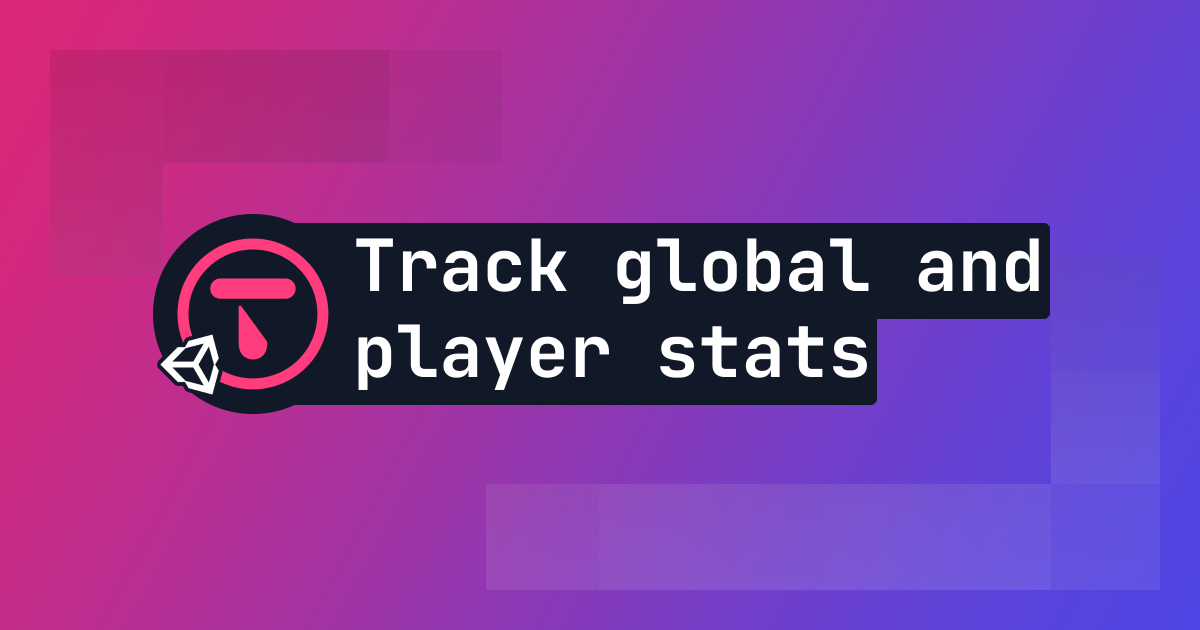
TL;DR
Talo Stats are a powerful way to track player actions in your Unity game. With just one line of code, you can track any stat. You can see global stats or inspect individual player stats in the Talo dashboard.
What is stat tracking?
Stat tracking involves taking a handful of key metrics in your game and updating them in response to player actions. Stat tracking can be really useful for understanding your players or even for showing-off recap information in blog posts.
After we've finished doing a game jam, we like to do a wrap-up post focusing on some of the stats from our game. During our most recent jam, we worked on a dystopian farming game where you drive around a modded combine harvester. Your combine harvester can take damage from any angle apart from the left flank, where it has a shield that blocks all damage.
To understand how well players understood the mechanic, we tracked the amount of damage blocked as well as the amount of damage taken. In this post, we'll go over how to do that.
Stat tracking through Talo
Talo provides an easy way to create stats and view them directly in the dashboard. The stat system provides a number of useful constraints to make sure players aren't attempting to cheat and your code is working as expected.
You can use Talo in any modern Unity game by simply importing the package through the Unity package manager.
Setup
Once you've loaded or created a project, go to the package manager (by navigating to Window > Package Manager
). Click the plus symbol and select "Add package from git url". When prompted, enter the url: https://github.com/TaloDev/unity-package.git
.
We've now added the Talo package to our Unity project. Doing this through git allows you to keep Talo up to date without needing to redownload the zip file and importing it.
Next, if you don't already have a Resources
folder, create one inside the Assets
folder. This folder allows you to load assets at run-time through code. Inside this folder, right-click and select Create > Talo > Settings Asset
.
If you click into this Asset, you'll find a number of configuration options. The two important ones are API url
(which you can change if you're choosing to self-host Talo) and Access key
which identifies your game. You'll need to create an access key through the Talo dashboard.
Generating an access key
Visit the Talo dashboard, login or create an account (and confirm your email address). Once logged in, create a new game (if you need to) and visit the Access Keys page.
Choose the scopes available to your access key (you'll need the read:players
, write:players
, read:gameStats
and write:gameStats
scopes) and create your access key. Copy your newly-generated access key into the Access key
field on the Settings Asset.
Creating a stat
To create your new stat visit the Stats page in the dashboard. You can also access it from the Services
dropdown. Configure your stat however you like but keep note of your chosen Internal name
since you'll need this to track your stat.
Identifying the player
We need to know which player to associate with which stat change. Let's create a script to do that automatically for us. Start off by creating a new GameObject in your scene and call it something like Identity Controller
.
Attach a script to the new GameObject, again I'm calling mine IdentityController
:
using UnityEngine;
using TaloGameServices;
using System;
public class IdentityController : MonoBehaviour
{
private async void Awake()
{
var id = PlayerPrefs.GetString("talo-id", Guid.NewGuid().ToString());
await Talo.Players.Identify("custom", id);
PlayerPrefs.SetString("talo-id", id);
}
}
We're using Unity's PlayerPrefs to load and save a random ID and associate it with that player. PlayerPrefs provides a fallback option in case there's no matching key (talo-id
in this example). This will happen the first time the player loads the game but going forward we'll use their assigned ID.
To identify a player we need to provide an identity service (this can be Steam, Epic, etc) - in this case we're using custom
to simply denote the randomised ID.
Incrementing stat(s)
Earlier I mentioned I wanted to track two things:
- Damage blocked by players (with an
Internal name
ofdamage-blocked
) - Damage taken by players (with an
Internal name
ofdamage-taken
)
Currently, I've got a bullet script that handles collision events through the OnTriggerEnter2D
callback:
private void OnTriggerEnter2D(Collider2D collision)
{
if (collision.CompareTag("Cockpit"))
{
collision.gameObject.GetComponent<Cockpit>().OnHit();
Remove();
else if (collision.CompareTag("Shield"))
{
Remove();
}
}
The player in my game is made up of two BoxColliders
. One is the Shield
where the player can't take damage and the other is the Cockpit
where the player can take damage.
Adding stat tracking to this script is really simple and can be done in just 2 lines of code:
using TaloGameServices;
private async void OnTriggerEnter2D(Collider2D collision)
{
if (collision.CompareTag("Cockpit"))
{
collision.gameObject.GetComponent<Cockpit>().OnHit();
Remove();
await Talo.Stats.Track("damage-taken");
else if (collision.CompareTag("Shield"))
{
Remove();
await Talo.Stats.Track("damage-blocked");
}
}
Note: we've had to mark this function as async
since we need to await
the tracking.
The Track()
function requires one parameter which is the Internal name
of your stat. You can optionally provide a second parameter which is the amount to increase/decrease the stat by.
Wrapping up
If you've ever wondered about how many players are performing specific actions, you can use Talo to easily track these actions. With just 1 line of code, we can track any stat and keep it constrainted within the limits you created it with.
Best of all, these stats are easily visible in the dashboard - you can see global stats or inspect players' individual stats too.
Build your game faster with Talo
Don't reinvent the wheel. Integrate leaderboards, stats, event tracking and more in minutes.
Using Talo, you can view and manage your players directly from the dashboard. It's free!
Get started
More from the Talo Blog
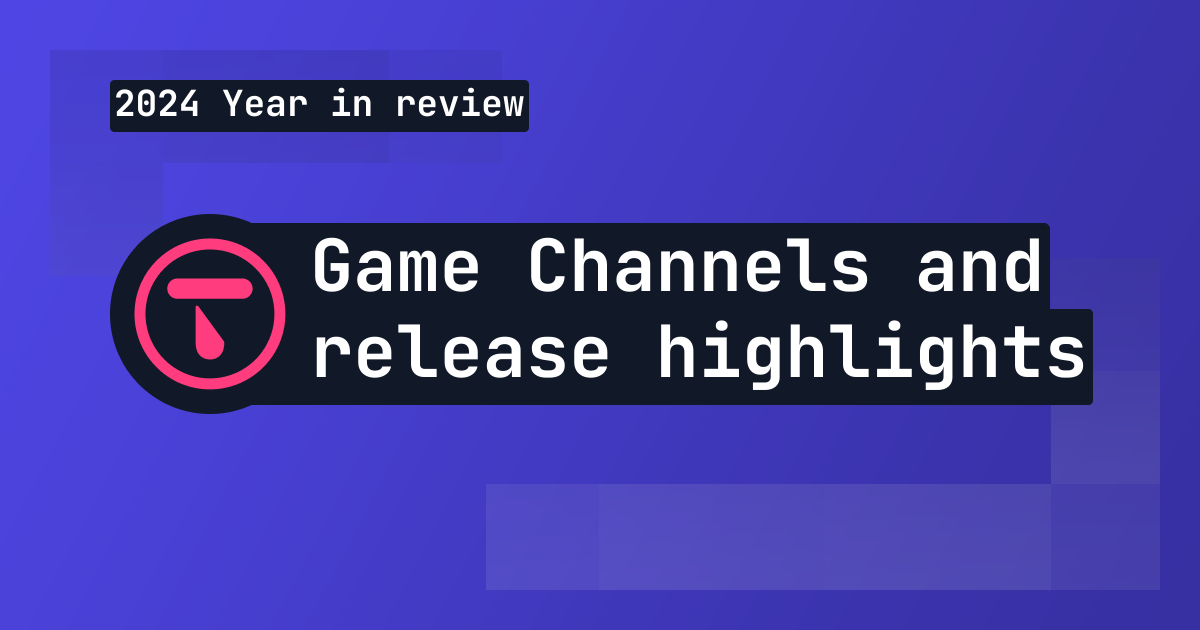
2024 Year in review: release highlights from Talo
A look back at Talo’s biggest updates in 2024, including game feedback, player auth, group functionality and channels.
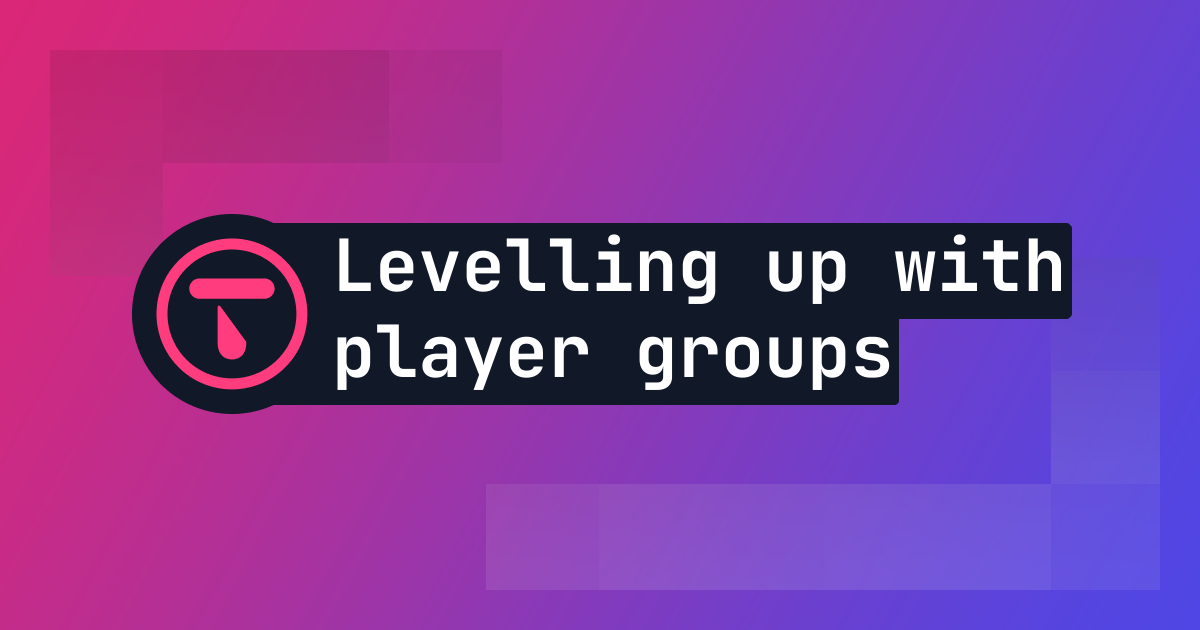
Levelling up your game with Talo’s Player Groups
Learn more about all the ways to segment your players and understand them better with Talo’s powerful group filtering system.
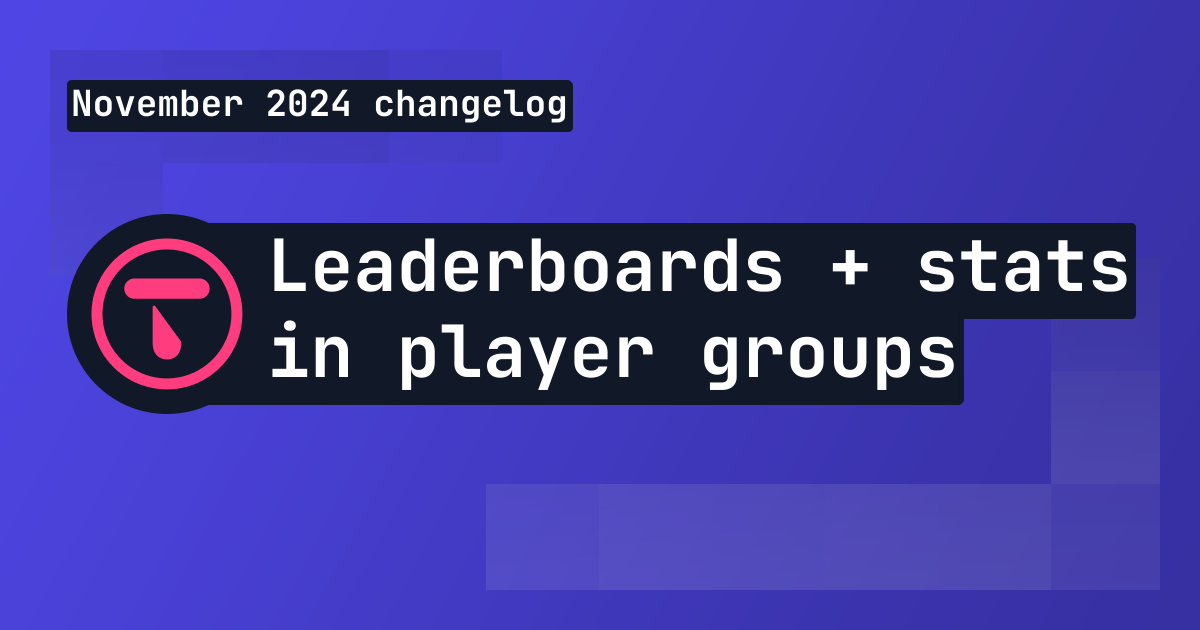
Changelog: New group filtering + interactive Playground
Catch up on Talo’s November 2024 updates, including a more interactive Playground, new group functionality and arm64 support.
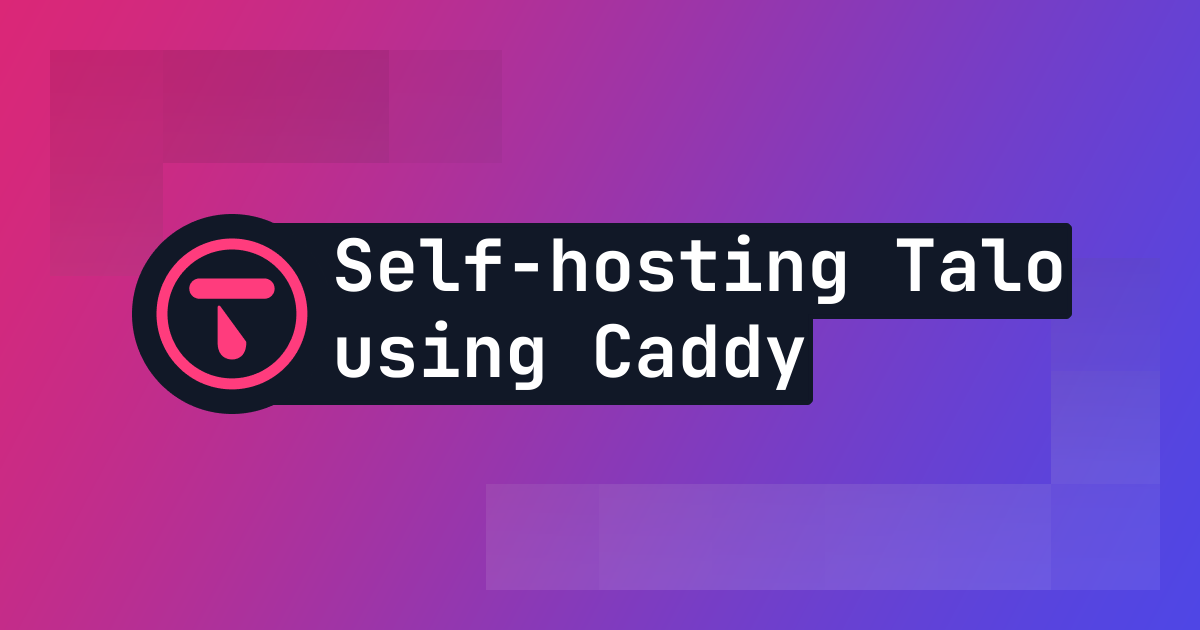
Exploring Talo’s new Caddy self-hosting template
A breakdown of Talo’s latest Caddy-based self-hosting option, plus a look at other self-hosting templates available for your game.