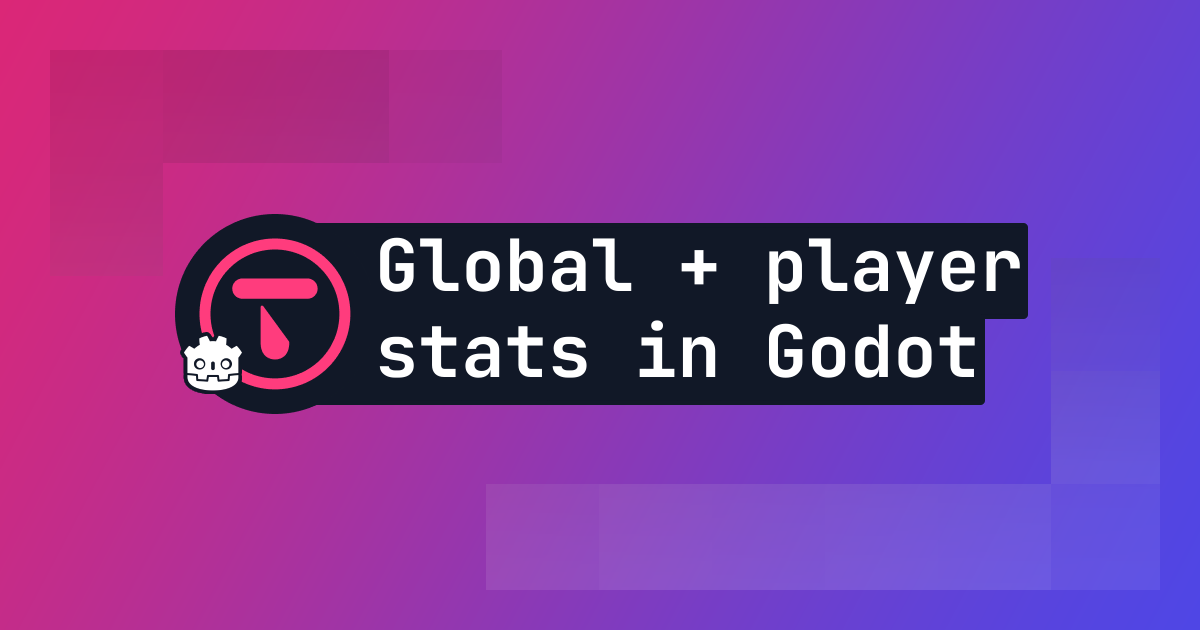
TL;DR
- Track individual player stats and global game metrics with minimal code
- Create competitive and collaborative mechanics using stat history
- Enable constraints and limits to prevent stat manipulation
Understanding Talo's game stats
Game statistics help you track what players are doing in your game - from how many enemies they've defeated to which levels they complete most often. Talo's Stats API lets you implement this tracking with just a few lines of code, saving you from building your own backend system or wrestling with database queries.
The Stats API includes comprehensive history tracking and analysis tools. You can fetch detailed stat histories for both individual players and global metrics:
# Get player's stat history
var history := await Talo.stats.get_history("boss_defeats")
for snapshot in history:
print("Defeated boss at: %s" % snapshot.created_at)
# Get global statistics
var global_stats := await Talo.stats.get_global_history("total_playtime")
print("Average playtime: %s" % global_stats.average_value)
Individual player tracking
Tracking individual player stats is incredibly straightforward. Let's say you want to track how many enemies a player has defeated:
# Track when a player defeats an enemy
var enemies_defeated := await Talo.stats.track("enemies_defeated")
print("Total enemies defeated: ", enemies_defeated.value)
The real power comes from the constraints you can apply to stats. You can set minimum and maximum values, limit how quickly stats can change and even specify default values:
# Example stat configuration
# - Minimum value: 0
# - Maximum value: 100
# - Maximum change per update: 10
# - Default value: 50
var gold_collected := await Talo.stats.track("gold_collected", -5) # Errors if the player goes below 0 gold
Talo provides built-in controls to maintain game balance, in the Talo dashboard you can:
- Set minimum and maximum values for stats
- Control update frequency with rate limiting
- Define maximum change amounts to prevent exploits
- Track creation and update timestamps
Global stats and community insights
What makes Talo's stat system particularly powerful is the ability to mark stats as global. This automatically aggregates values across all players, giving you insights into community-wide achievements:
# Get global statistics for levels completed
# You could also filter this by date to, for example, see how many levels were completed this week
var level_stats := await Talo.stats.get_global_history("levels_completed")
print("Average levels completed: ", level_stats.global_value.average_value)
print("Highest levels completed: ", level_stats.global_value.max_value)
Creating competitive mechanics
The stat history system enables powerful competitive mechanics, particularly for time-based challenges. Here's how you can implement a weekly championship in a boss-rush-style game:
# Get global boss defeat stats for the last week
var end_time = Time.get_unix_time_from_system()
var start_time = end_time - (7 * 24 * 60 * 60) # 7 days ago
# In the real world you would check for additional pages from this paginated API
var boss_stats := await Talo.stats.get_global_history("bosses_defeated", 0, start_time, end_time)
# Find the player with the highest boss defeats
var champion = null
var highest_value := 0
for snapshot in boss_stats.history:
if snapshot.value > highest_value:
highest_value = snapshot.value
champion = snapshot
if champion and champion.player_id == current_player.id:
award_weekly_champion_rewards()
This historical data system opens up plenty of possibilities for competitive features like:
- Monthly competitions
- Seasonal player versus player rankings
- Daily challenges for roguelike games
Collaborative gameplay features
Stats aren't just for competition - they can drive collaborative gameplay too. By tracking global progress, you can create community goals and shared achievements:
# Track a contribution to a community goal
var res := await Talo.stats.track("community_resources_gathered", 10.0)
print("Global progress: %s" % res.stat.global_value)
if res.stat.global_value >= 1000:
unlock_community_reward()
# Tip: you could also award a random player using the pick_random() array function
Talo's easy to use Stats API allows you to think about features like:
- Community progression where player contributions unlock new areas or items
- Global events that track collective achievements (e.g. boss defeats during a specific week)
- Shared resource pools that everyone can contribute to
Getting started with Talo stats
Ready to implement powerful stat tracking in your game? Here's how to get started:
- Install the Talo Godot plugin
- Configure your stats in the dashboard
- Start tracking with just a few lines of code
Visit the stats feature page to learn more about what's possible with Talo's stat system and check out the Godot stat tracking docs for a deeper dive into the APIs highlighted in this post.
Join our growing community of game developers on Discord to share ideas, get help and see how others are using Talo's stats system in their games.
Conclusion
The Talo Stats API is robust and flexible enough to work in every kind of game. You can build competitive features or collaborative challenges without needing to worry about or manage any of your own stat tracking infrastructure.
Whether you're tracking individual achievements or player-wide global stats, Talo's stat system takes care of all the complicated logic and lets you focus on building your game.
Build your game faster with Talo
Don't reinvent the wheel. Integrate leaderboards, stats, event tracking and more in minutes.
Using Talo, you can view and manage your players directly from the dashboard. It's free!
Get started
More from the Talo Blog
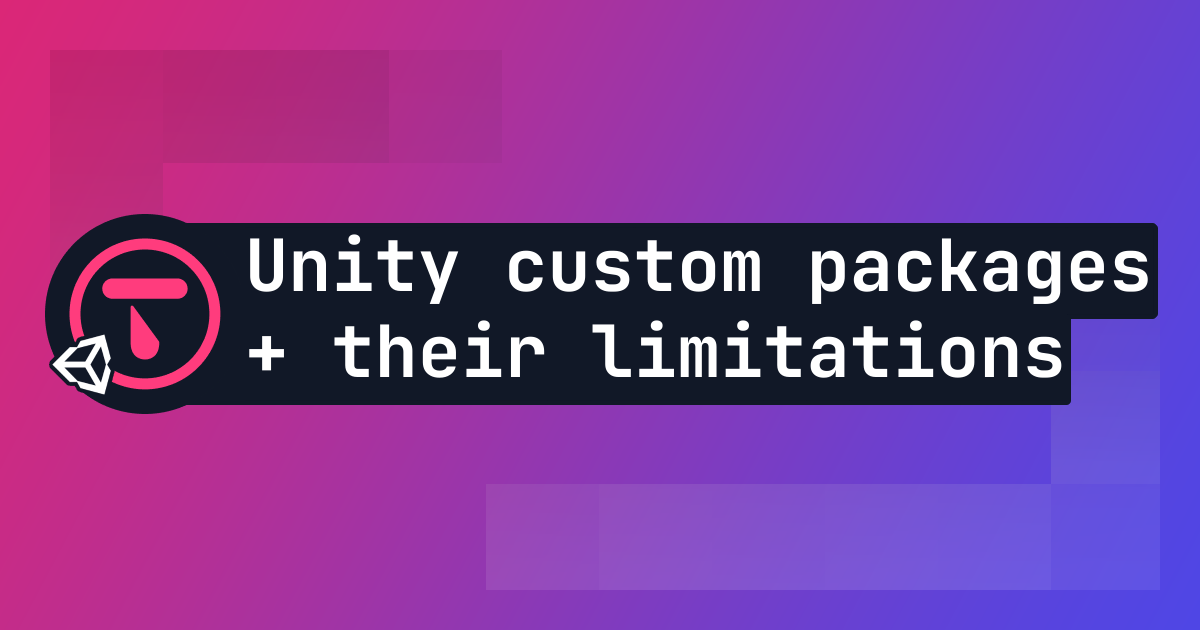
How to create a custom Unity package - and why you shouldn't
Thinking of creating a custom Unity package? Learn the process, common pitfalls, and why dependency management is a major challenge.
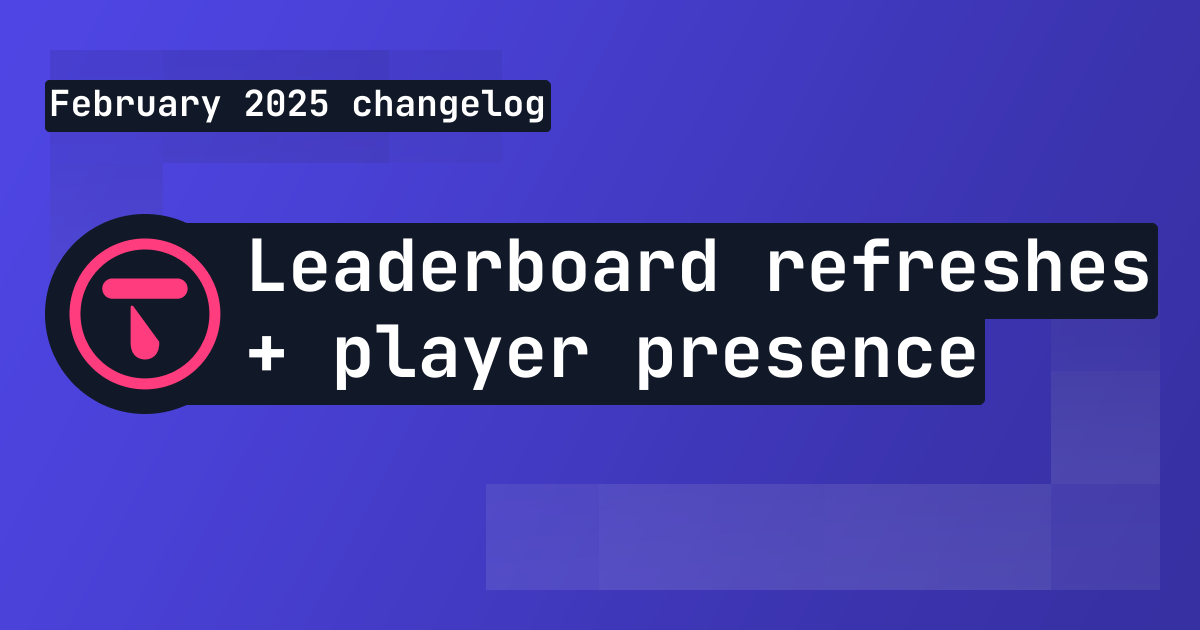
Changelog: leaderboard refreshes and player presence
This month we've added support for daily leaderboard refreshes and a new API for player presence tracking.
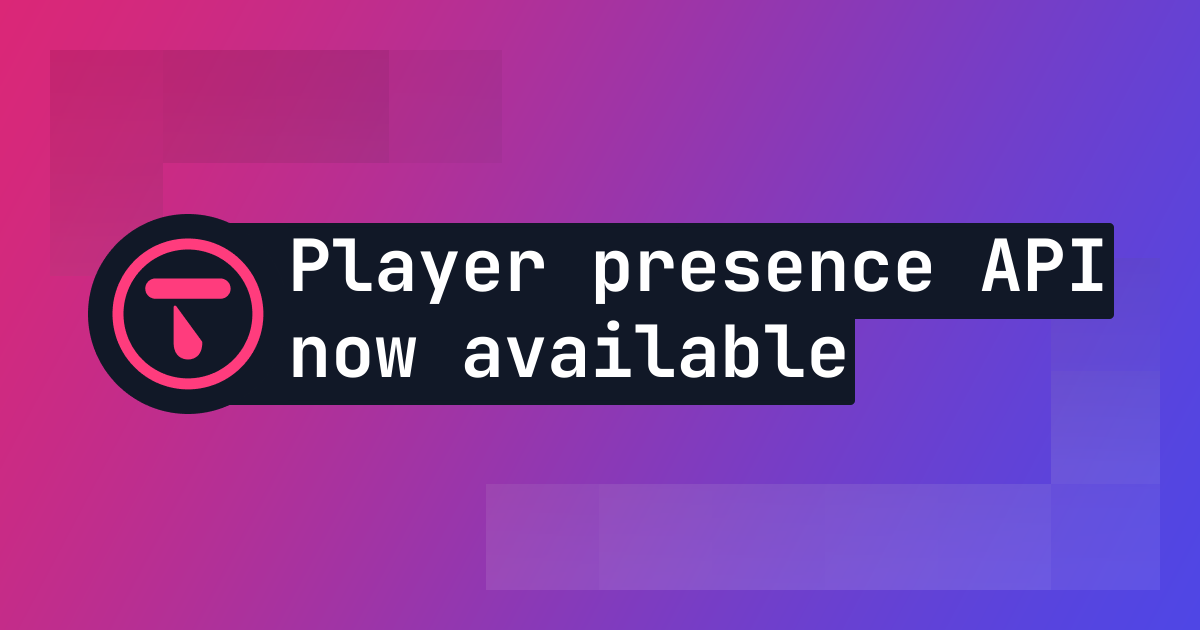
Introducing the Talo Presence API: real-time player tracking made easy
Learn how to implement real-time player presence tracking in your game with Talo's new Presence API. Easily track online status, custom states and build social features.
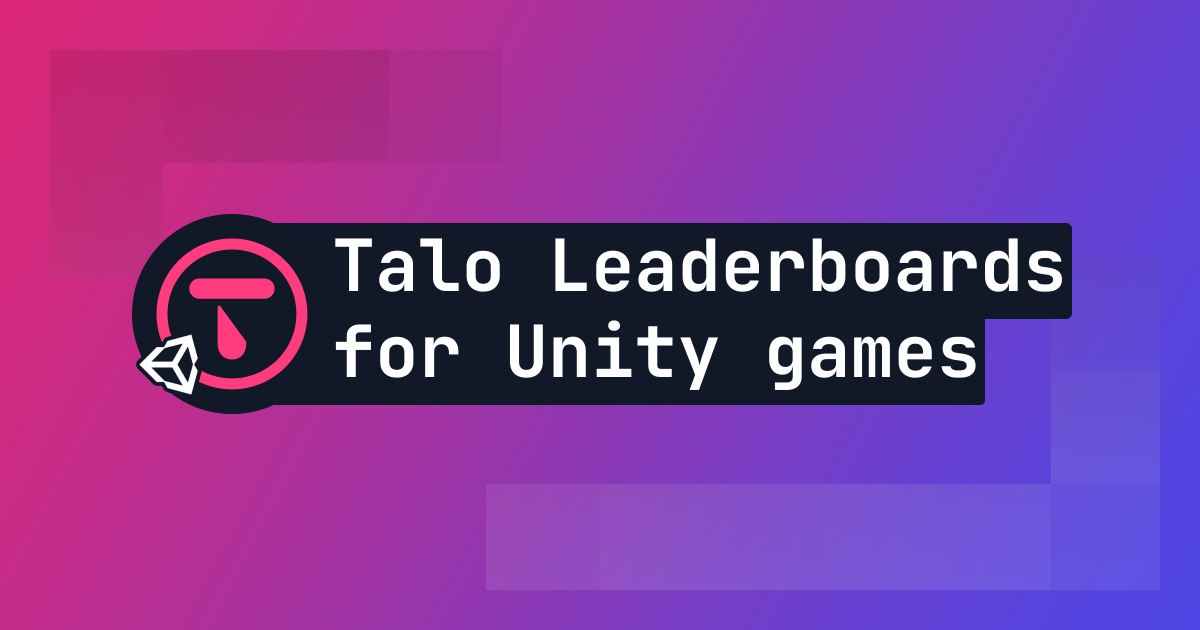
Level up your Unity game with Talo Leaderboards
From daily leaderboards to custom properties and filtering, Talo provides the most comprehensive leaderboards for Unity games.