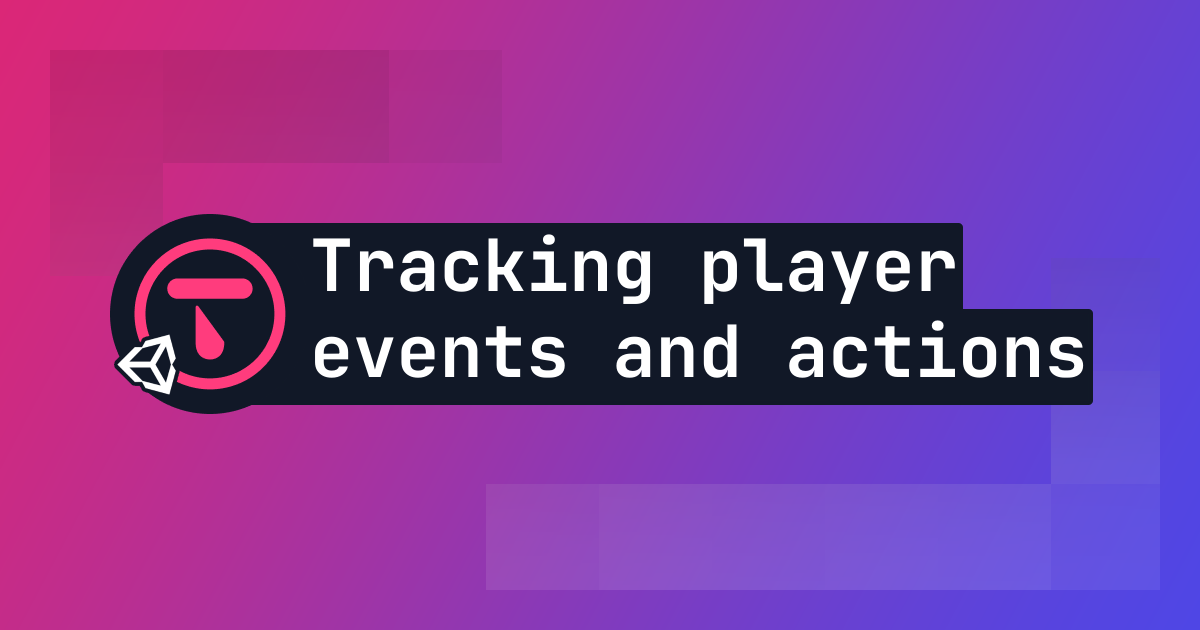
TL;DR
Using Talo, you can track player events in your Unity game with just one line of code. This allows you to see how players are interacting with your game and what parts they're engaging with the most.
What is event tracking?
Sometimes how your players play your game can be a bit of a black box. Unless your players are being vocal about exactly what they're doing on, for example, Discord, you probably don't know how they're interacting with the various parts of your game.
This is where event tracking comes in - you can declaratively choose what to track and when it gets tracked. You could track when a player levels up or when a player completes a level. You can even send extra metadata about that event such as what level they have progressed to.
Event tracking through Talo
With just one line of code, you can track any event in your game using Talo. The Talo dashboard lets you then see and compare events as well as drill-down into a specific player's events to debug tricky situations. See the Talo events overview page for more details on how it all works.
You can use Talo in any modern Unity game by simply importing the package through the Unity package manager.
Setup
Once you've loaded or created a project, go to the package manager (by navigating to Window > Package Manager
). Click the plus symbol and select "Add package from git url". When prompted, enter the url: https://github.com/TaloDev/unity-package.git
.
We've now added the Talo package to our Unity project. Doing this through git allows you to keep Talo up to date without needing to redownload the zip file and importing it.
Next, if you don't already have a Resources
folder, create one inside the Assets
folder. This folder allows you to load assets at run-time through code. Inside this folder, right-click and select Create > Talo > Settings Asset
.
If you click into this Asset, you'll find a number of configuration options. The two important ones are API url
(which you can change if you're choosing to self-host Talo) and Access key
which identifies your game. You'll need to create an access key through the Talo dashboard.
Generating an access key
Visit the Talo dashboard, login or create an account (and confirm your email address). Once logged in, create a new game (if you need to) and visit the Access Keys page.
Choose the scopes available to your access key (you'll need the read:players
, write:players
and write:events
scopes) and create your access key. Copy your newly-generated access key into the Access key
field on the Settings Asset.
Identifying the player
We need to know which player is sending events. Let's create a script to do that automatically for us. Start off by creating a new GameObject in your scene and call it something like Identity Controller
.
Attach a script to the new GameObject, again I'm calling mine IdentityController
:
using UnityEngine;
using TaloGameServices;
using System;
public class IdentityController : MonoBehaviour
{
private async void Awake()
{
var id = PlayerPrefs.GetString("talo-id", Guid.NewGuid().ToString());
await Talo.Players.Identify("custom", id);
PlayerPrefs.SetString("talo-id", id);
}
}
We're using Unity's PlayerPrefs to load and save a random ID and associate it with that player. PlayerPrefs provides a fallback option in case there's no matching key (talo-id
in this example). This will happen the first time the player loads the game but going forward we'll use their assigned ID.
To identify a player we need to provide an identity service (this can be Steam, Epic, etc) - in this case we're using custom
to simply denote the randomised ID.
Sending event data
Tracking events is incredible easy. All it takes is one line of code:
Talo.Events.Track("Cast spell");
Since we've identified the player we know this data belongs to them, so all we need to do is pass through the name of the event we want to track.
In the real world you'll usually want to track extra properties with your events. For example, let's track what spells players are casting:
using TaloGameServices;
public class PlayerController : MonoBehaviour
{
public int primarySpellID;
public int spellLevel;
private void Update()
{
if (Input.GetMouseButtonDown(0))
{
CastSpell();
}
}
private void CastSpell()
{
// TODO: logic for casting the spell
Talo.Events.Track(
"Cast spell",
("Spell ID", $"{primarySpellID}"),
("Spell level", $"{spellLevel}")
);
}
}
As you can see, to capture extra event properties we can simply pass in as many string tuples as we like to the Track()
function.
Avoiding event tracking pitfalls
The main issues you'll have with event tracking will both stem from not being consistent:
- Make sure you name your events consistently (for example
(Verb) (object)
- e.g.Cast spell
) - Avoid dynamic event names - for example
Cast [spell name] spell
. Instead, keep your events generic and send a consistent property with the name of the spell.
Additionally, make sure you're only tracking meaningful events so that you don't get overwhelmed when searching through all your game's events. Avoid events that could get sent too often, for example a Damage taken
event if your game has weapons that rapidly trigger damage effects.
Wrapping up
Event tracking lets you get rid of the guess-work. By tracking important actions when they happen, you're able to gauge how well a mechanic is being used and even track the overall progress of your players. See how often potions get used, items get bought and how many level-ups there are per day, month or year.
Talo lets you seamlessly start tracking events with just one line of code. Using the Talo dashboard you can visualise these events and even see which players are sending them.
Build your game faster with Talo
Don't reinvent the wheel. Integrate leaderboards, stats, event tracking and more in minutes.
Using Talo, you can view and manage your players directly from the dashboard. It's free!
Get started
More from the Talo Blog
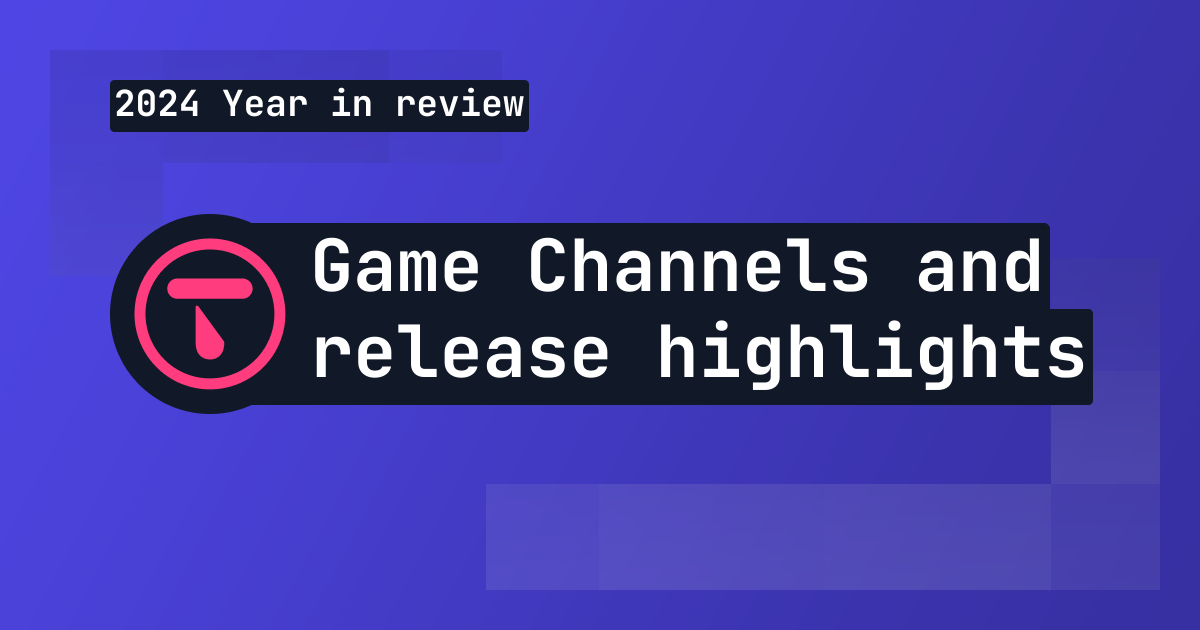
2024 Year in review: release highlights from Talo
A look back at Talo’s biggest updates in 2024, including game feedback, player auth, group functionality and channels.
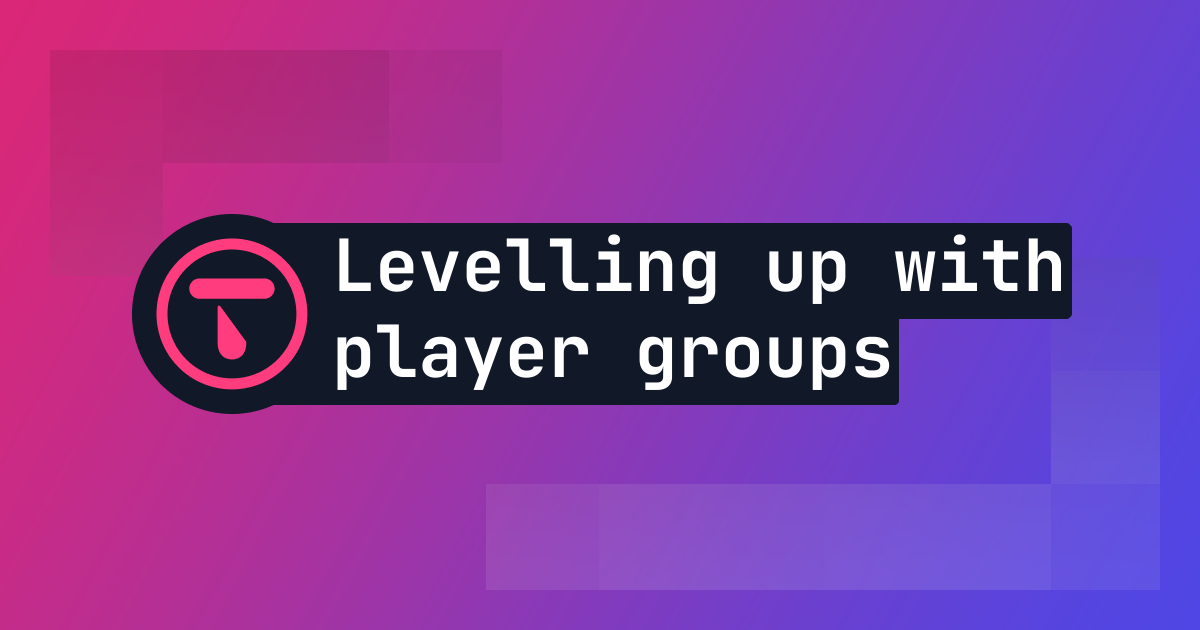
Levelling up your game with Talo’s Player Groups
Learn more about all the ways to segment your players and understand them better with Talo’s powerful group filtering system.
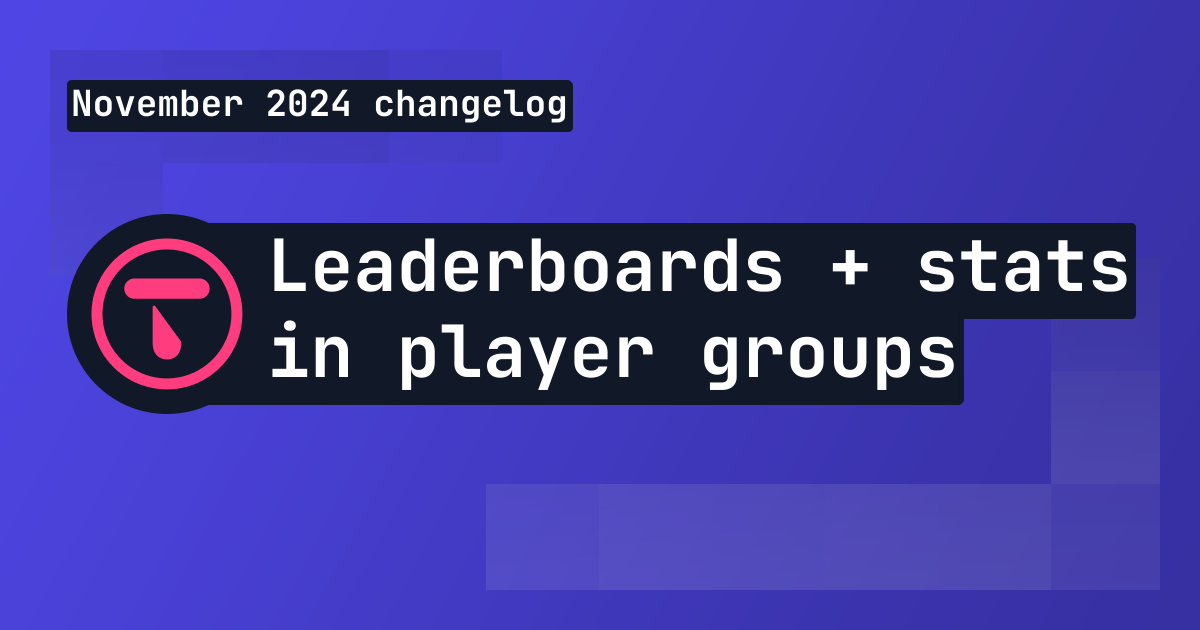
Changelog: New group filtering + interactive Playground
Catch up on Talo’s November 2024 updates, including a more interactive Playground, new group functionality and arm64 support.
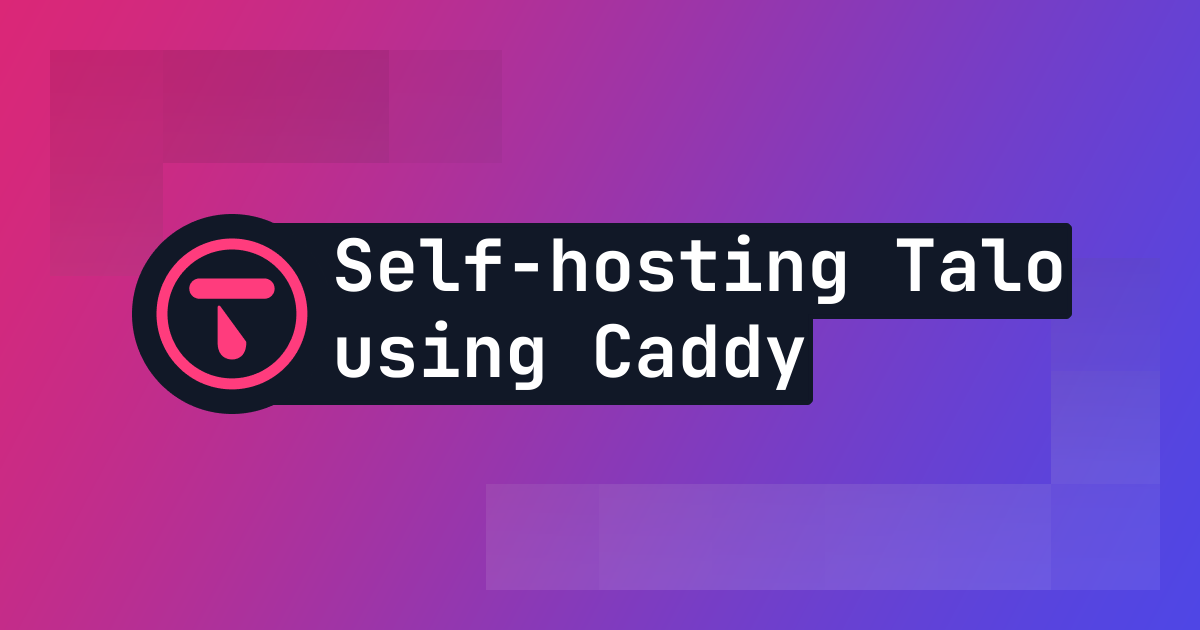
Exploring Talo’s new Caddy self-hosting template
A breakdown of Talo’s latest Caddy-based self-hosting option, plus a look at other self-hosting templates available for your game.